Image Rollovers
The image rollover is a very common feature found throughout the web. It is an effect where an image (the primary image) will change to a different (secondary) image when the user moves their mouse over the image link. Then the image usually changes back to the original (primary) image when the user moves the mouse away from the image link. It is a simple effect to accomplish, and relatively easy to "install". Below is a quick example for you to hover your mouse over. All the links on this page do not
do anything, they are just for example:
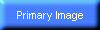
See, nothing special, I'm sure you've seen this type of thing numerous times before. That example had the normal purple border which usually surrounds a link image. If you do not want the border, you simply set the
border to
"none" in the image tag (
<img src="img.jpg" border="none">). The next example is basically the same thing except the border has been removed:
To set up an image swapping script, you'll have to do a few things:
- Add a few lines of Javascript code in the HEAD section of your HTML page which will perform the actual image swapping:
<script language="javascript">
function swapImage(imgN,imgU){
if(document.images)document.images[imgN].src=imgU;
}
</script>
The swapImage() function simply tests if the browser supports the document.images object (needed for image swapping), and if so, swaps the named image with the new image URL. You'll see how this is used in steps 4 and 5.
- Create an image tag and "wrap" it in a link tag. Don't forget to add the border="none" part unless you want the purple link border around the image. Example:
<a href="linkURL">
<img src="img1.jpg" border="none">
</a>
- Assign the image a unique name value. This is important! If you have two images with the same name value, the script may cause errors or not work at all. This name will be used so the script knows which image you want to access. This name can be whatever you want it to be, I like to use text followed by a number value to make it easy to keep track. eg: image1, image2, image3, etc. Example:
<a href="linkURL">
<img src="img1.jpg" border="none" name="image1">
</a>
- Add an onmouseover event handler and a function call to make the browser swap the image when the user hovers over the image link. You have to pass the image name and the secondary image URL you want to swap to the function. Example:
<a href="linkURL" onmouseover="swapImage( 'image1' , 'img2.jpg' )">
<img src="img1.jpg" border="none" name="image1">
</a>
- Add an onmouseout event handler and a function call to make the browser swap the original image back when the user's mouse leaves the image link. If you forget this part, the image won't change back to whatever it was before you hovered over it. You have to pass the image name and the primary image URL you want to swap to the function. Example:
<a href="linkURL" onmouseover="swapImage( 'image1' , 'img2.jpg' )" onmouseout="swapImage( 'image1' , 'img1.jpg' )">
<img src="img1.jpg" border="none" name="image1">
</a>
In steps 4 and 5, you may have noticed the onmouseover event handlers were enclosed in double quotes, while the parameters for the function were enclosed in single quotes. This is done on purpose and is called quote nesting. Both types of quotes do basically the same thing, but you have to follow this format or you'll wind up with weird errors and it might not be easy to figure out why. Just make sure you have an end quote for every single or double quote.
Here is a working 2-button example along with the code used to create it. Parts of the code have been highlighted to show the most important sections.
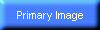
|
<a href="#0" onmouseover="swapImage('i4','../images/img2.jpg' )" onmouseout="swapImage('i4','../images/img1.jpg' )" > <img src="img1.jpg" name="i4" border="none" > </a>
<a href="#0" onmouseover="swapImage('i5','../images/img2.jpg' )" onmouseout="swapImage('i5','../images/img1.jpg' )" > <img src="img1.jpg" name="i5" border="none" > </a>
|
Now, the code above works well,
except there would be a delay as the user hovers over the image. Why? Because when you hover over the image, the browser has to load the replacement image from the server. This delay might be negligible with a fast Internet connection, but we must keep our 56k (and lower) users in mind. :)
To fix this, we can simply add a little extra Javascript code to
codeload, or
cache, all the secondary images.
You do not have to codeload the primary images since the browser has already loaded them when it initially rendered the page.
The highlighted section below is the extra code that handles the codeloading of the images.
<script language="javascript">
function codeLoadImages(){
var t='<layer top="-100" left="0" visibility="hide"><div style="position:absolute; top:-100px; left:0px; visibility:hidden">';
for(i=0;i<arguments.length;i++)t+='<img src="'+arguments[i]+'" height="10" width="10">';
t+='</div></layer>';
document.write(t);
}
codeLoadImages('img2.jpg');
function swapImage(imgN,imgU){
if(document.images)document.images[imgN].src=imgU;
}
</script>
Simply call the
codeLoadImages() function and pass the image names to codeload. Don't forget to wrap them in single quotes and separate multiple entries with commas. Since I am only using one secondary image on this page (even though there are multiple instances of that image), there is only one image to codeload. An example to load several (fictional) images is:
codeLoadImages('imageA.jpg', 'pic2.gif', 'img23.gif', 'images/background.gif');
For those who want to know what is going on here; the
codeLoadImages() simply creates an absolutely-positioned element and adds all the image tags inside it. However, this element is hidden from view and placed off the top of the page so it will never be seen. There is another method to codeload images, but I find this is the most reliable since the image you are codeloading will actually reside on the page, so you
know it will be loaded.
Hopefully this tutorial was able to help you to create simple and effective image rollover effects for your page.